Development and Test Environment
These examples have been tested on a machine with JDK 1.8 version and developed at Eclipse IDE for Java EE Developers (Oxygen 2). Download the installation here and choose Eclipse IDE for Java EE Developers to install it on your computer.
Creating a Java Project in Eclipse
This chapter explains the steps creating a new Java project.
Run “Eclipse”.
Go to “File” menu.
Select "New" - “Java Project”.
Enter a project name "CWS" and click the “Finish” button.
A new project will be created with the given name.
Setting a Web Service Client up
This chapter explains the steps setting up a web service client in your new Java project.
Click mouse right button on the project name under the Package Explorer.
Click the [New] - [Other ...].
Enter “Web Service” at wizards input box.
Select “Web Service Client“, then click “Next” button.
Enter http://e3.sap.cubemaster.net/calculation.svc?singlewsdl to the “Service definition” input box.
Define the “Develop client” as shown in the screen shot.
Then click the “Finish” button.
New packages and classes will be created automatically.

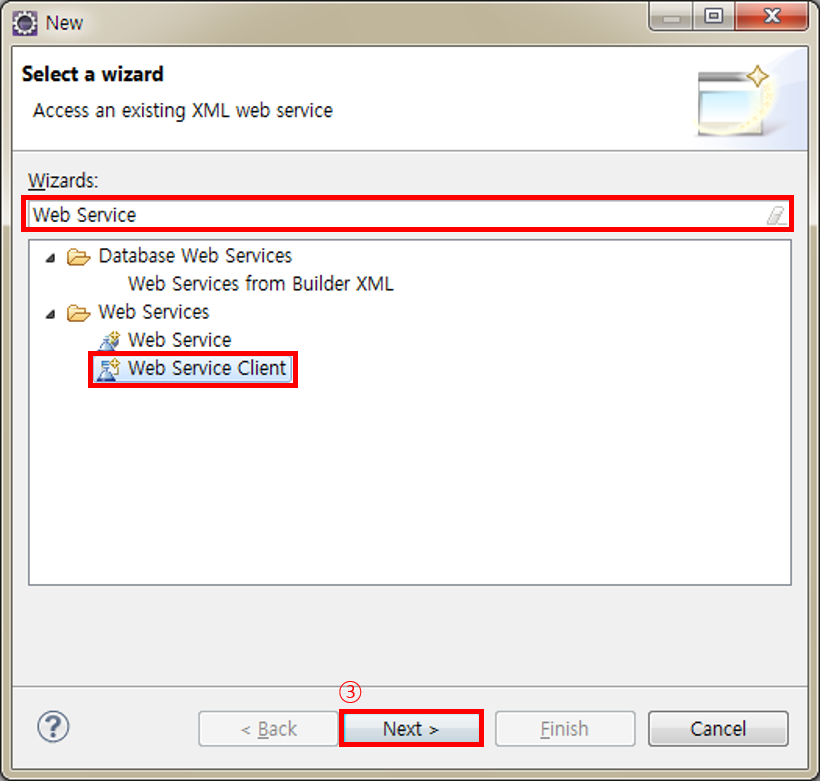
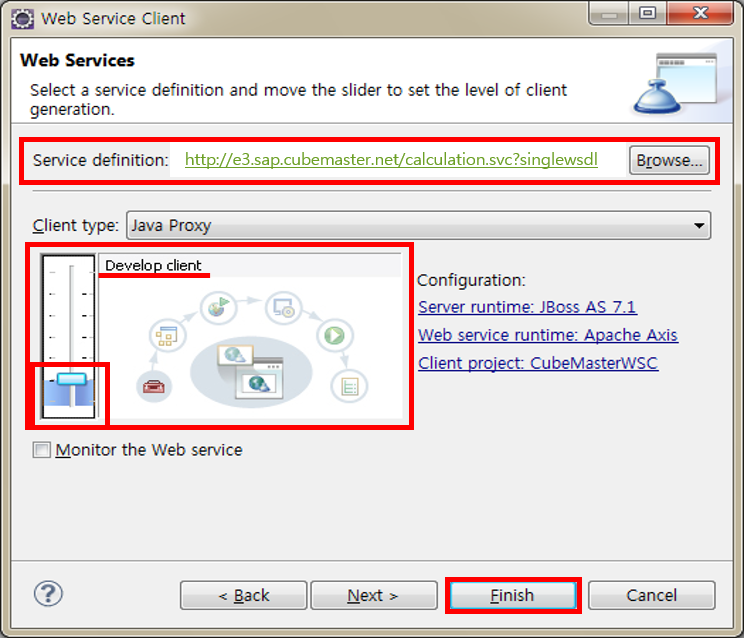
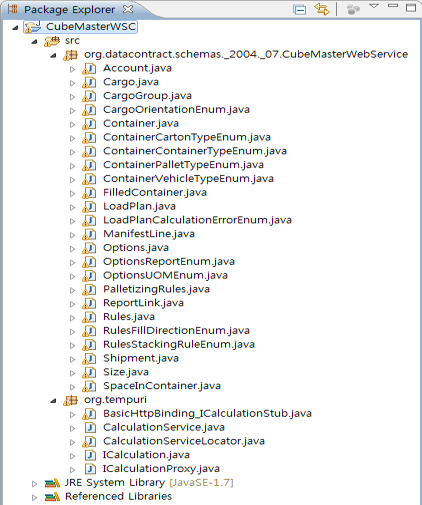
Adding a Main Class Package and Class
Click the mouse right button on “scr” package.
Click the “Package” on popup menu.
Enter Package name “org.main” at Name input box.Go to “org.main” package and then click the mouse right button.
Click the [New]-[Class].
Enter class name at name input box.
Check automatically method .
Select public static void main(String[] args).
Then click “Finish” button.
Main class will be generated automatically as screen shot.
Firstly, import all packages(Package Name org.tempuri.*,org.datacotract.schemas.*).
Write a try-catch statement in the main method.
Editing the Main Class Package and Class
Main class will be generated automatically as screen shot.
package org.main;
import org.tempuri.*;
import java.io.ByteArrayInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.apache.axis.encoding.Base64;
import org.datacontract.schemas._2004._07.CubeMasterWebService.*;
public class LoadBuildingSample {
public static void main(String[] args) {
try {
} catch (Exception e) {
// TODO Auto-generated catch block
}
}
}
Import all packages (Package Name org.tempuri.*,org.datacotract.schemas.*).
Write a try-catch statement in the main method.
Add new statements to define the shipment information for the calculation. Define “Account”, “Shipment”, and “Options”. Define two cargoes. Define “Container” and “Shipment” rules.
Add new statements to send the shipment information to the CubeMaster service servers.
Add a try-catch statements to look up the results by seeing if “getStatus()” returns “OK”.
Add new statements to produce an image in a try-catch statement from the results.
Add cleanup statements.
package org.main;
import org.tempuri.*;
import java.io.ByteArrayInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import org.apache.axis.encoding.Base64;
import org.datacontract.schemas._2004._07.CubeMasterWebService.*;
public class LoadBuildingSample {
public static void main(String[] args) {
// TODO Auto-generated method stub
try {
ICalculationProxy iCalculationProxy = new ICalculationProxy();
//Define account information
//Please replace with the information of your account
Account account = new Account();
account.setUserID("chang@logen.co.kr");
account.setPassword("****");
account.setCompany("Logen Solutions");
//Define options
Options options = new Options();
options.setCalculationSaved(true);
options.setGraphicsCreated(true);
options.setGraphicsImageWidth((short) 300);
options.setGraphicsImageDepth((short) 300);
options.setThumbnailsCreated(true);
options.setThumbnailsImageWidth((short) 300);
options.setThumbnailsImageDepth((short) 300);
options.setUOM(1);//1=mm+Kg, 0=inch+lbs, 2=Cm+Kg
//Define shipment
Shipment shipment = iCalculationProxy.getShipment();
shipment.setTitle("New Shipment Sample for Vehicle Load v2");
shipment.setDescription("in Java Eclipse");
//Define two cargoes
Cargo[] Cargoes = new Cargo[3];
Cargoes[0] = new Cargo();
Cargoes[0].setName("First Cargo");
Cargoes[0].setLength(500);//mm
Cargoes[0].setWidth(500);//mm
Cargoes[0].setHeight(500);//mm
Cargoes[0].setQty((long) 40);
Cargoes[0].setSequence(1);
Cargoes[0].setGroupName("B");
Cargoes[0].setWeight(47.12);//kg
Cargoes[0].setColorKnownName("BLUE");
Cargoes[0].setFloorStackSupportsOthers(true);
Cargoes[1] = new Cargo();
Cargoes[1].setName("Second Cargo");
Cargoes[1].setLength(700);//mm
Cargoes[1].setWidth(800);//mm
Cargoes[1].setHeight(700);//mm
Cargoes[1].setQty((long) 156);
Cargoes[1].setSequence(2);
Cargoes[1].setGroupName("A");
Cargoes[1].setWeight(50.00);//kg
Cargoes[1].setColorKnownName("RED");
Cargoes[1].setFloorStackSupportsOthers(true);
Cargoes[2] = new Cargo();
Cargoes[2].setName("Third Cargo");
Cargoes[2].setLength(600);//mm
Cargoes[2].setWidth(550);//mm
Cargoes[2].setHeight(860);//mm
Cargoes[2].setQty((long)45);
Cargoes[2].setSequence(2);
Cargoes[2].setGroupName("A");
Cargoes[2].setWeight(12.00);//kg
Cargoes[2].setOrientationsAllowed(63);
Cargoes[2].setColorKnownName("YELLOW");
//Cargoes[2].setColorHexaCode("#FF00FF");
Cargoes[2].setFloorStackSupportsOthers(false);
shipment.setCargoes(Cargoes);
//Define containers
Container[] EmptyContainers = new Container[2];
EmptyContainers[0] = new Container();
EmptyContainers[0].setContainerType(2);//2=SeaContainer
EmptyContainers[0].setName("20FT Dry");
EmptyContainers[0].setLength((double) 5890);//mm
EmptyContainers[0].setWidth((double) 2330);//mm
EmptyContainers[0].setHeight((double) 2380);//mm
EmptyContainers[0].setMaxWeight((double) 5000);//kg
EmptyContainers[1] = new Container();
EmptyContainers[1].setContainerType(2);//2=SeaContainer
EmptyContainers[1].setName("40FT Dry");
EmptyContainers[1].setLength((double) 12050);//mm
EmptyContainers[1].setWidth((double) 2330);//mm
EmptyContainers[1].setHeight((double) 2380);//mm
EmptyContainers[1].setMaxWeight((double) 7000);//kg
shipment.setContainers(EmptyContainers);
//Define Rules
shipment.getRules().setIsWeightLimited(true);
shipment.getRules().setIsSequenceUsed(false);
shipment.getRules().setIsGroupUsed(false);
shipment.getRules().setIsSafeStackingUsed(true);
shipment.getRules().setMinSupportRate((double) 60);
shipment.getRules().setStackingRule(5);
shipment.getRules().setFillDirection(1);
//Run the calculation
LoadPlan loadPlan = iCalculationProxy.run(shipment, account, options);
//Show the return
System.out.println(loadPlan.getStatus());
//Show an error code if any
System.out.println(loadPlan.getCalculationError());
if(loadPlan.getStatus().equals("OK")) {
//Access the results
System.out.println("# of Containers =" + loadPlan.getFilledContainers().length);
InputStream is = null;
OutputStream os = null;
//Show all filled containers
try {
for (FilledContainer container : loadPlan.getFilledContainers()) {
//show the name of the container
System.out.println(container.getName());
System.out.println(container.getLength());
} // End of foreach - FilledContainer
} catch (Exception e) {
// TODO: handle exception
} finally { //Resource Cleanup
if(is != null) {
try {
is.close();
os.close();
} catch(IOException e) {
e.printStackTrace();
}
}
} // end of try-catch statement .. for Show all filled containers
} //LoadPlan.Status == "OK"
System.exit(0);
} catch (Exception e) {
// TODO: handle exception
}
}
}
I am typically in order to running a blog and that i actually appreciate your content. The actual article has actually peaks my personal interest. I am going to save your web site and gaze after checking for brand spanking new data. https://boktv11.com
Everyone should consider the 랜덤추첨기 for their next event
The workshops listed on the 대외활동 사이트 are top-notch
I love the thrill of using 꽁머니 on new games.
There are innumerable blogs in the world of the internet. But trust me, this site contains every single detail that makes it exceptional. I'll return time and time again. 네팔 시민을 위한 캄보디아 비자
Discover the world-class education at Seoul Scholars International’s 국제학교
블랙툰 is my go-to site for discovering new webtoons
발산노래방 offers an incredible karaoke experience with its top-notch audio system and vast song selection. A must-visit for karaoke lovers!
툰코 has become my favorite way to enjoy webtoons
Enjoying free webtoons is so easy with 웹툰 무료보기
Finding great webtoons on 웹툰 미리보기 has been so easy. I love the site’s layout
The 웹툰 다시보기 webtoon site is great for keeping up with all the new releases
Daily episodes and diverse genres make 뉴토끼 a webtoon fan’s paradise
Can’t stop reading the latest romance series on 블랙툰
비툰’s daily updates are perfect for keeping up with ongoing webtoons. Love it
The webtoons on 툰코 주소 are always so engaging and well-drawn
블랙툰 대체 is great, but expanding your horizons with different webtoon sites can enhance your overall reading enjoyment.
블랙툰 has the best webtoons I’ve ever read. Highly recommend checking it out
Really enjoying the interactive community on 툰코. Great way to connect with other fans
The 뉴토끼 webtoon site is fantastic for finding fresh content daily